Core audio tips
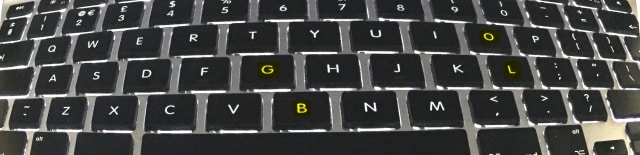
Incase it ever gets removed, some Core audio gold from http://www.subfurther.com/blog/2009/04/28/an-iphone-core-audio-brain-dump/
- The Audio Unit Programming Guide is required reading for using Audio Units, though you have to filter out the stuff related to writing your own AUs with the C++ API and testing their Mac GUIs.
- Get comfortable with pointers, the address-of operator (
&
), and maybe evenmalloc
. - You are going to fill out a lot of
AudioStreamBasicDescription
structures. It drives some people a little batty. - Always clear out your ASBDs, like this:
memset(&myASBD, 0, sizeof (myASBD));
This zeros out any fields that you haven’t set, which is important if you send an incomplete ASBD to a queue, audio file, or other object to have it filled in.
- Use the “canonical” format — 16-bit integer PCM — between your audio units. It works, and is far easier than trying to dick around bit-shifting 8.24 fixed point (the other canonical format).
- Audio Units achieve most of their functionality through setting properties. To set up a software renderer to provide a unit with samples, you don’t call some sort of a setRenderer() method, you set the
kAudioUnitProperty_SetRenderCallback
property on the unit, providing aAURenderCallbackStruct
struct as the property value. - Setting a property on an audio unit requires declaring the “scope” that the property applies to. Input scope is audio coming into the AU, output is going out of the unit, and global is for properties that affect the whole unit. So, if you set the stream format property on an AU’s input scope, you’re describing what you will supply to the AU.
- Audio Units also have “elements”, which may be more usefully thought of as “buses” (at least if you’ve ever used pro audio equipment, or mixing software that borrows its terminology). Think of a mixer unit: it has multiple (perhaps infinitely many) input buses, and one output bus. A splitter unit does the opposite: it takes one input bus and splits it into multiple output buses.
- Don’t confuse buses with channels (ie, mono, stereo, etc.). Your ASBD describes how many channels you’re working with, and you set the input or output ASBD for a given scope-and-bus pair with the stream description property.
- Make the RemoteIO unit your friend. This is the AU that talks to both input and output hardware. Its use of buses is atypical and potentially confusing. Enjoy the ASCII art:
------------------------- | i o | -- BUS 1 -- from mic --> | n REMOTE I/O u | -- BUS 1 -- to app --> | p AUDIO t | -- BUS 0 -- from app --> | u UNIT p | -- BUS 0 -- to speaker --> | t u | | t | -------------------------
Ergo, the stream properties for this unit are
Bus 0 | Bus 1 | |
Input Scope: | Set ASBD to indicate what you’re providing for play-out | Get ASBD to inspect audio format being received from H/W |
Output Scope: | Get ASBD to inspect audio format being sent to H/W | Set ASBD to indicate what format you want your units to receive |
- That said, setting up the callbacks for providing samples to or getting them from a unit take global scope, as their purpose is implicit from the property names:
kAudioOutputUnitProperty_SetInputCallback
andkAudioUnitProperty_SetRenderCallback
. - Michael Tyson wrote a vital blog on recording with RemoteIO that is required reading if you want to set callbacks directly on RemoteIO.
- Apple’s
aurioTouch
example also shows off audio input, but is much harder to read because of its ambition (it shows an oscilliscope-type view of the sampled audio, and optionally performs FFT to find common frequencies), and because it is written with Objective-C++, mixing C, C++, and Objective-C idioms. - Don’t screw around in a render callback. I had correct code that didn’t work because it also had
NSLog
s, which were sufficiently expensive that I missed the real-time thread’s deadlines. When I commented out theNSLog
, the audio started playing. If you don’t know what’s going on, set a breakpoint and use the debugger. - Apple has a convention of providing a “user data” or “client” object to callbacks. You set this object when you setup the callback, and its parameter type for the callback function is
void*
, which you’ll have to cast back to whatever type your user data object is. If you’re using Cocoa, you can just use a Cocoa object: in simple code, I’ll have a view controller set the user data object asself
, then cast back toMyViewController*
on the first line of the callback. That’s OK for audio queues, but the overhead of Obj-C message dispatch is fairly high, so with Audio Units, I’ve started using plain C structs. - Always set up your audio session stuff. For recording, you must use
kAudioSessionCategory_PlayAndRecord
and callAudioSessionSetActive(true)
to get the mic turned on for you. You should probably also look at the properties to see if audio input is even available: it’s always available on the iPhone, never on the first-gen touch, and may or may not be on the second-gen touch. - If you are doing anything more sophisticated than connecting a single callback to RemoteIO, you may want to use an AUGraph to manage your unit connections, rather than setting up everything with properties.
- When creating AUs directly, you set up a
AudioComponentDescription
and use the audio component manager to get the AUs. With an AUGraph, you hand the description toAUGraphAddNode
to get back the pointer to anAUNode
. You can get the Audio Unit wrapped by this node withAUGraphNodeInfo
if you need to set some properties on it. - Get used to providing pointers as parameters and having them filled in by function calls:
AudioUnit remoteIOUnit; setupErr = AUGraphNodeInfo(auGraph, remoteIONode, NULL, &remoteIOUnit);
Notice how the return value is an error code, not the unit you’re looking for, which instead comes back in the fourth parameter. We send the address of the remoteIOUnit
local variable, and the function populates it.
- Also notice the convention for parameter names in Apple’s functions.
inSomething
is input to the function,outSomething
is output, andioSomething
does both. The latter two take pointers, naturally. - In an AUGraph, you connect nodes with a simple one-line call:
setupErr = AUGraphConnectNodeInput(auGraph, mixerNode, 0, remoteIONode, 0);
This connects the output of the mixer node’s only bus (0) to the input of RemoteIO’s bus 0, which goes through RemoteIO and out to hardware.
- AUGraphs make it really easy to work with the mic input: create a RemoteIO node and connect its bus 1 to some other node.
- RemoteIO does not have a gain or volume property. The mixer unit has volume properties on all input buses and its output bus (0). Therefore, setting the mixer’s output volume property could be a de facto volume control, if it’s the last thing before RemoteIO. And it’s somewhat more appealing than manually multiplying all your samples by a volume factor.
- The mixer unit adds amplitudes. So if you have two sources that can hit maximum amplitude, and you mix them, you’re definitely going to clip.
- If you want to do both input and output, note that you can’t have two RemoteIO nodes in a graph. Once you’ve created one, just make multiple connections with it. The same node will be at the front and end of the graph in your mental model or on your diagram, but it’s OK, because the captured audio comes in on bus 1, and some point, you’ll connect that to a different bus (maybe as you pass through a mixer unit), eventually getting the audio to RemoteIO’s bus 0 input, which will go out to headphones or speakers on bus 0.