Creating a parent-child object heirarchy to display in tree view
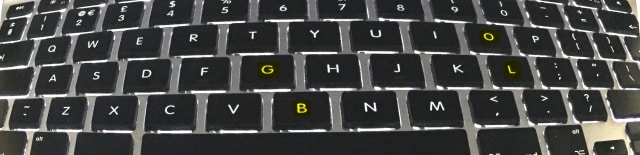
I had a module that required display of a set of categories organised by id and parent id. To create a tree view I created a node object and small bit of code to render a HTML drop down form element.
Of course after creating this code I realised this information was in fact surplus to requirements and would potentially confuse users.
The code took too much love to delete so here it is for next time…
class UKOLCatTree { public $id; public $parentid; public $title; public $children; public $level; function __construct($data) { $this->id = $data['id']; $this->parentid = $data['parent_id']; $this->title = $data['title']; $this->children = array(); $this->level = 0; } function addChild($cat) { array_push($this->children, $cat); $cat->level = $this->level + 1; } function show() { $this->showSelf(); foreach ($this->children as $kids) { $kids->show(); } } function showSelf() { echo ''; for ($i = 0; $i level; $i++) { echo " "; echo " "; if ($i == ($this->level - 1)) { echo "|_"; } else { echo ". "; } } echo $this->title . '[' . $this->id . '-' . $this->parentid . ']
'; } function getOptionHTML() { $r = $this->getSelfOptionHTML(); foreach ($this->children as $kids) { $r .= $kids->getOptionHTML(); } return $r; } function getSelfOptionHTML() { $r = 'id . '">'; for ($i = 0; $i level; $i++) { $r .= " "; if ($i == ($this->level - 1)) { $r .= "|_"; } else { $r .= ". "; } } $r .= $this->title . '' . "n"; return $r; } } $db =& JFactory::getDBO(); $db->setQuery('SELECT * FROM `#__categories` WHERE section="com_docman"'); $root = new UKOLCatTree(array('id' => 0, 'parent_id' => 0, 'title' => 'Top')); $cats = array($root); $rows = $db->loadAssocList(); foreach ($rows AS $row) { $cat = new UKOLCatTree($row); $cats[$cat->id] = $cat; } foreach ($cats AS $cat) { if (isset($cats[$cat->parentid])) { if ($cat->id != 0) { $dad = $cats[$cat->parentid]; $dad->addChild($cat); } } else { echo 'No parent [' . $cat->parentid . '] for cat [' . $cat->id . ']
'; } } echo $root->getOptionHTML();